Inheritance – gtest and gmock part 1
- Czester16
- 8 cze 2017
- 2 minut(y) czytania
Hi everyone!
Here I want to show more tips about programming. We end basics, but not all just that much as you need to start. So in 5 post I will show you basic knowledge for corporation c++ linux/embeded developer basic. They have often TTD way of work, but tests are important so even in smaller companies you can use those knowledge.
So today I will write more about connections between objects. Can you imagine basic structure for our quite new cRPG system? We want to have warrior and archer, but they all are people. To put them to one table and use similar we need to know few things the basic connections between them, so we want to create basic class.
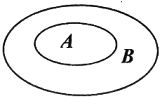
So we can start from talking about basic functionality of basic class. We need: move, attack, know position and ect. I divide it into groups public and protected, because private part of class is not available for child objects. Can be important for us how many peoples we create in our world from any reasons – it can be our counter of players which not died yet in our game. Pure virtual function determine abstract class like now. Sometimes you can heard word interface – it is class where every method is virtual (has no body) and don’t have variables. Interfaces are more popular in java and C#, there interface is language keyword. Pragma once defend us, because we should load code just one time, even if two files are trying to load it more than one time to memory (warior.h, archer.h). Let’s code.
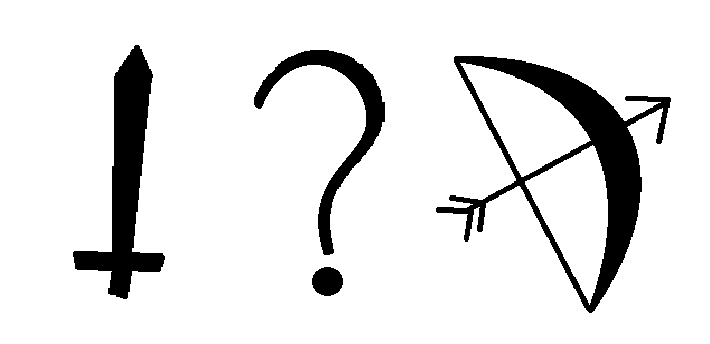
human.h
#pragma once
class human
{
public:
virtual void move(int)=0;
virtual void attack(human*)=0;
int getPosition();
int getHealth();
void getHit(int);
static int getCounter();
protected:
int health = 100;
int position = 0;
static int counter;
static void incrementCounter();
};
warior.h
#pragma once
#include "human.h"
class warior : public human
{
public:
warior();
~warior();
void move(int);
void attack(human*);
};
archer.h
#pragma once
#include "human.h"
class archer : public human
{
public:
archer();
~archer();
void move(int);
void attack(human*);
};
We need to create body for those classes, so make it fast and we have our simple structure for characters.
human.cc
#include "human.h"
int human::counter = 0;
int human::getPosition()
{
return position;
};
int human::getHealth()
{
return health;
};
void human::getHit(int hitPower)
{
health -= hitPower;
}
int human::getCounter()
{
return counter;
};
void human::incrementCounter()
{
counter = getCounter()+1;
};
warior.cc
#include "warior.h"
warior::warior()
{
}
warior::~warior()
{
}
void warior::move(int steps)
{
position += steps;
}
void warior::attack(human* man)
{
man->getHit(10);
}
archer.cc
#include "archer.h"
archer::archer()
{
}
archer::~archer()
{
}
void archer::move(int steps)
{
position += steps;
}
void archer::attack(human* man)
{
man->getHit(10);
}
And voila we have simple classes which inherits from the human class. In the next blog we try to make simple menu for program and secondly we will try to compile it.
Comments