Compile complex project – gtest and gmock part 2
- Czester16
- 11 cze 2017
- 2 minut(y) czytania
Hi, hey, hello! Second part is coming…
Ok on the way to awesomeness we can make few short code for menu and then we will try to compile it. So we can start from code of main file.
main.cc
#include <iostream>
#include "archer.h"
#include "warrior.h"
human *player;
void createwarrior() {
warrior warriorPlayer;
player = &warriorPlayer;
}
void createArcher() {
archer archerPlayer;
player = &archerPlayer;
}
int main() {
char choice;
std::cout << "Chose your character. Write a (for archer) or w (for warrior)." << std::endl;
std::cin >> choice;
switch(choice){
case 'a':
archer();
std::cout << "Archer is good choice. Have a nice day!" << std::endl;
break;
case 'w':
warrior();
std::cout << "warrior is strong man. Have a nice day!" << std::endl;
break;
default:
std::cout << "Meh, weak joke... Bye!" << std::endl;
break;
}
}
So we create a class which depend on human choice. We have our player which can have two classes based on human class. We can do it because inherited classes contain an inherited object like here:
You can imagine that we have pointer to A object (human) which is inside object B. Program has no sense of course, but we created an object and we can compile it now.
Compiling need to be divided for parts:
Compiling,
Linking.
We need to use compiling flags, because we used C++11 standard and we don’t want to have wall of warnings.
Compile it all:
g++ -c -Wall -std=c++11 -o human.o human.cc
g++ -c -Wall -std=c++11 -o archer.o archer.cc
g++ -c -Wall -std=c++11 -o warrior.o warrior.cc
g++ -c -Wall -std=c++11 -o main.o main.cc
Now we have to link it, because we have dependencies between files:
G++ -Wall -std=c++11 -o MyGame warrior.o archer.o human.o main.o
What we exactly made? We create few compiled object and secondly we link it together inside one program. During next part we start to automatize process of compilation using make.
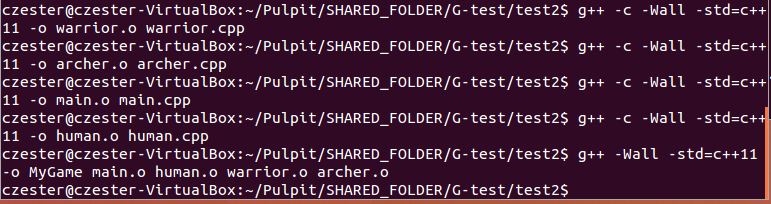
Commenti